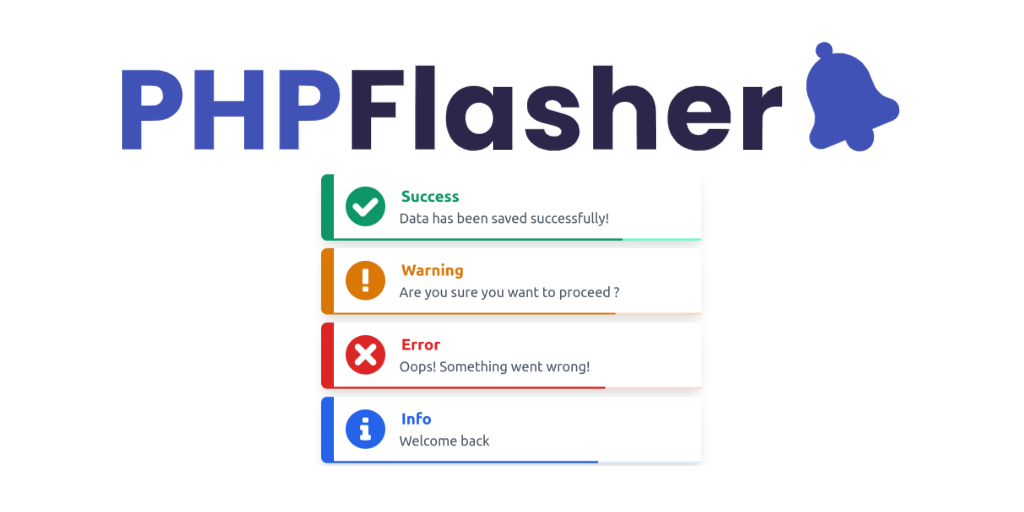
Integrating PHPFlasher with Laravel: A Complete Guide for Beginners
Laravel vs. PHPFlasher
Laravel
- Static alert takes up valuable page space
- Requires manual template implementation
- No auto-dismissal functionality
PHPFlasher
- Elegant toast notification that preserves layout
- Zero template modifications required
- Auto-dismissal with visual countdown
Adding flash notifications to your Laravel application becomes incredibly straightforward with PHPFlasher. In this complete guide, you'll learn everything you need to know to get started with PHPFlasher v2 in your Laravel projects.
Why Use PHPFlasher in Laravel?
Laravel does come with a built-in flash messaging system through its session, but it has several limitations:
- Limited Display Options: Laravel's default flash messages are just stored in the session - you need to build your own UI components.
- No Toast Notifications: You need additional JavaScript libraries to show toast-style notifications.
- Manual Integration: You have to manually integrate front-end code with backend session data.
- Inconsistent Experience: Different parts of your application might handle notifications differently.
What is PHPFlasher?
PHPFlasher is a powerful, easy-to-use flash notification library for PHP. It provides a simple, unified API to create beautiful toast notifications in your web applications.
Key Features
- Framework-agnostic with dedicated Laravel integration
- Beautiful toast notifications without any extra CSS
- Consistent API across different notification types
- Support for multiple notification libraries
Why Developers Love It
- Single line of code for beautiful notifications
- Zero configuration needed to get started
- Works seamlessly with all modern Laravel versions
- Extensive customization when you need it
PHPFlasher v2
The latest version includes improved performance, enhanced styling options, and better framework integrations. It's the perfect time to start using PHPFlasher in your projects!
Getting Started in Seconds
First, install PHPFlasher using Composer. The process is extremely simple:
composer require php-flasher/flasher-laravel
Zero Configuration
That's it! No service provider registration, no publishing assets, no template modifications. PHPFlasher v2 auto-registers and injects everything you need.
Now you can create beautiful notifications with a single line of code:
// In your controller
public function store(Request $request)
{
// Process the form submission
$user = User::create($request->validated());
// Flash a success message
flash()->success('User created successfully!');
return redirect()->route('users.index');
}
No Template Changes Needed
With PHPFlasher v2, you don't need to add any
@flasher_render
directive to your templates. All required assets are automatically injected into your HTML.
Comparing Laravel Flash vs. PHPFlasher
Let's compare the traditional Laravel approach with PHPFlasher to see the difference in implementation and results:
Laravel
In your controller:
// Controller
public function update(Request $request, $id)
{
$user = User::findOrFail($id);
$user->update($request->validated());
session()->flash('message', 'User updated successfully!');
session()->flash('alert-type', 'success');
return redirect()->route('users.index');
}
In your Blade template:
@if (session('message'))
<div class="alert alert-">
</div>
@endif
With PHPFlasher
In your controller:
// Controller
public function update(Request $request, $id)
{
$user = User::findOrFail($id);
$user->update($request->validated());
flash()->success('User updated successfully!');
return redirect()->route('users.index');
}
In your Blade template:
// Nothing needed! PHPFlasher v2 auto-injects everything
Notification Types
PHPFlasher supports different notification types out of the box. Here are the main types you'll use:
Success Notification
Used to indicate successful operations
flash()->success('Changes saved successfully!');
Error Notification
Used to indicate errors or failures
flash()->error('Something went wrong!');
Warning Notification
Used for important warnings
flash()->warning('You are about to delete this item.');
Info Notification
Used for general information
flash()->info('New features are available.');
Customizing Notifications
PHPFlasher provides a fluent interface for customizing your notifications. Here are some common customizations:
flash()
->position('bottom-right') // Change position
->timeout(5000) // Set timeout in milliseconds (5 seconds)
->success('Profile updated successfully!');
Position Options
top-left
top-center
top-right
bottom-left
bottom-center
bottom-right
Configuration
PHPFlasher works out of the box with default settings, but you can publish the configuration file to customize it:
php artisan vendor:publish --tag=flasher-config
This will create a config/flasher.php
file with the following structure:
<?php
declare(strict_types=1);
use Flasher\Prime\Configuration;
/*
* Default PHPFlasher configuration for Laravel.
*
* This configuration file defines the default settings for PHPFlasher when
* used within a Laravel application. It uses the Configuration class from
* the core PHPFlasher library to establish type-safe configuration.
*
* @return array PHPFlasher configuration
*/
return Configuration::from([
// Default notification library (e.g., 'flasher', 'toastr', 'noty', 'notyf', 'sweetalert')
'default' => 'flasher',
// Path to the main PHPFlasher JavaScript file
'main_script' => '/vendor/flasher/flasher.min.js',
// List of CSS files to style your notifications
'styles' => [
'/vendor/flasher/flasher.min.css',
],
// Set global options for all notifications (optional)
// 'options' => [
// 'timeout' => 5000, // Time in milliseconds before the notification disappears
// 'position' => 'top-right', // Where the notification appears on the screen
// ],
// Automatically inject JavaScript and CSS assets into your HTML pages
'inject_assets' => true,
// Enable message translation using Laravel's translation service
'translate' => true,
// URL patterns to exclude from asset injection and flash_bag conversion
'excluded_paths' => [],
// Map Laravel flash message keys to notification types
'flash_bag' => [
'success' => ['success'],
'error' => ['error', 'danger'],
'warning' => ['warning', 'alarm'],
'info' => ['info', 'notice', 'alert'],
],
// Set criteria to filter which notifications are displayed (optional)
// 'filter' => [
// 'limit' => 5, // Maximum number of notifications to show at once
// ],
// Define notification presets to simplify notification creation (optional)
// 'presets' => [
// 'entity_saved' => [
// 'type' => 'success',
// 'message' => 'Entity saved successfully',
// ],
// ],
]);
Key Configuration Options
Option | Description | Default |
---|---|---|
default |
The default notification library to use | flasher |
inject_assets |
Automatically inject assets into HTML pages | true |
translate |
Enable translation of notification messages | true |
options.timeout |
Default notification timeout (ms) | 5000 |
options.position |
Default notification position | top-right |
Advanced Usage
Using Multiple Notification Libraries
PHPFlasher supports multiple notification libraries. By default, it uses its own library, but you can also use other popular libraries like SweetAlert, Toastr, and Noty:
First, install the adapter:
# For SweetAlert
composer require php-flasher/flasher-sweetalert-laravel
# For Toastr
composer require php-flasher/flasher-toastr-laravel
# For Noty
composer require php-flasher/flasher-noty-laravel
Then use it in your code:
// Using SweetAlert
sweetalert()->success('This is a SweetAlert notification');
// Using Toastr
toastr()->info('This is a Toastr notification');
// Using Noty
noty()->warning('This is a Noty notification');
Troubleshooting Guide
Notifications Not Appearing
If your notifications aren't showing up, check these common issues:
-
-
-
excluded_paths
configuration
JavaScript Errors
If you're seeing JavaScript errors in the console:
Opening your browser's console (F12) will help identify any JavaScript errors.
Styling Issues
If notifications appear but don't look right:
Try adding !important
to your custom styles if you need to override defaults.
Best Practices
Do
- Keep messages clear and concise
- Match notification type to the context (success, error, etc.)
- Use longer timeouts for important messages
- Use consistent positioning throughout your app
- Consider translating messages for multilingual apps
Don't
- Overuse notifications for non-important actions
- Display multiple notifications at once
- Use lengthy messages that users won't read
- Mix notification types inappropriately
- Forget to handle errors and display appropriate notifications
Ready to enhance your Laravel app with beautiful notifications?
PHPFlasher provides an elegant, powerful solution for all your notification needs with minimal setup and maximum flexibility.
Conclusion
PHPFlasher simplifies the implementation of flash notifications in Laravel applications, making it easy to provide users with important feedback about their actions. With its intuitive API, beautiful out-of-the-box styling, and extensive customization options, PHPFlasher is the perfect solution for developers looking to enhance their application's user experience.
By following the examples and best practices outlined in this guide, you'll be well on your way to implementing a professional notification system that not only looks great but also provides clear and timely information to your users.