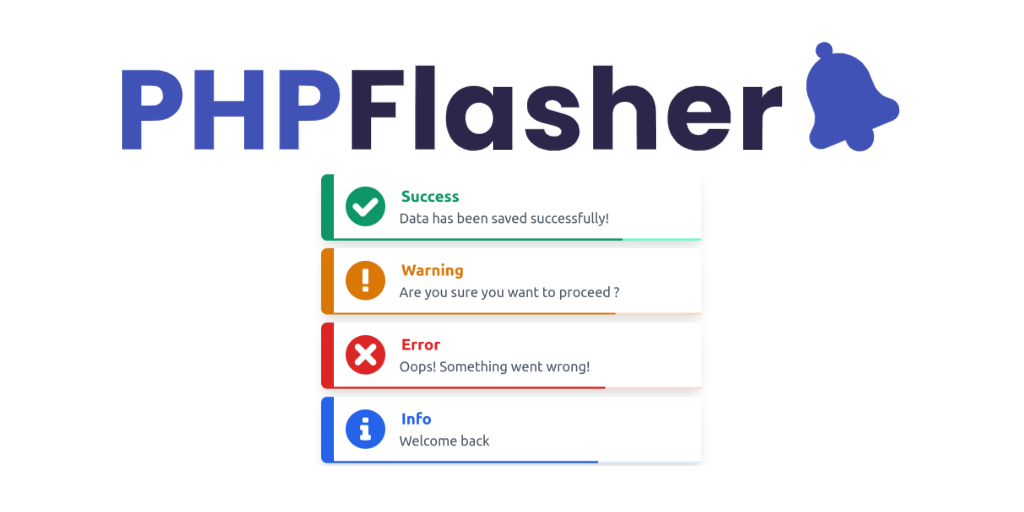
PHPFlasher Configuration Options Explained for Beginners
PHPFlasher v2 comes with a powerful configuration system that helps you customize your notifications to fit your application perfectly. In this beginner's guide, we'll break down each configuration option to help you understand what they do and how to use them.
Why Configuration Matters
While PHPFlasher works great with its default settings, understanding the configuration options gives you:
- Complete control over the appearance and behavior of your notifications
- Consistent styling across your entire application
- The ability to create reusable notification templates with presets
Getting Started with PHPFlasher Configuration
First, let's look at how to access and modify your PHPFlasher configuration. If you're using Laravel, you can publish the configuration file with:
php artisan vendor:publish --tag=flasher-config
This will create a config/flasher.php
file in
your project. Let's explore what's inside:
<?php
declare(strict_types=1);
use Flasher\Prime\Configuration;
/*
* Default PHPFlasher configuration for Laravel.
*/
return Configuration::from([
// Default notification library
'default' => 'flasher',
// Path to the main PHPFlasher JavaScript file
'main_script' => '/vendor/flasher/flasher.min.js',
// List of CSS files to style your notifications
'styles' => [
'/vendor/flasher/flasher.min.css',
],
// Other options...
]);
Core Configuration Options Explained
'default' Option
'default' => 'flasher',
This sets the default notification library PHPFlasher will use. Options include:
-
flasher
: The default built-in adapter -
toastr
: For Toastr-style notifications -
noty
: For Noty.js notifications -
sweetalert
: For SweetAlert notifications
'main_script' & 'styles' Options
'main_script' => '/vendor/flasher/flasher.min.js',
'styles' => [
'/vendor/flasher/flasher.min.css',
],
These options control the JavaScript and CSS files that PHPFlasher loads:
-
main_script
: Path to the main JavaScript file -
styles
: Array of CSS files to load
You can change these to use CDN links or custom paths if needed.
'options' Setting
// Set global options for all notifications
'options' => [
'timeout' => 5000,
'position' => 'top-right',
],
This setting defines default behavior for all notifications:
-
timeout
: Time in milliseconds before notifications disappear (5000 = 5 seconds) -
position
: Where notifications appear (top-right, top-left, bottom-right, bottom-left, etc.)
These global options can be overridden for individual notifications.
'inject_assets' & 'translate' Options
'inject_assets' => true,
'translate' => true,
These options control important PHPFlasher behaviors:
-
inject_assets
: Whentrue
, PHPFlasher automatically adds the required JavaScript and CSS to your pages -
translate
: Whentrue
, PHPFlasher will try to translate notification messages using your framework's translation system
Setting inject_assets
to false
means you'll need to manually include the required assets in your templates.
Advanced Configuration Options
'excluded_paths' Option
'excluded_paths' => [
'api/*',
'admin/reports/*',
],
This option lets you specify URL patterns where PHPFlasher should not:
- Inject JavaScript and CSS assets
- Convert framework flash messages to PHPFlasher notifications
This is useful for API routes or admin sections that don't need notifications.
'flash_bag' Option
'flash_bag' => [
'success' => ['success'],
'error' => ['error', 'danger'],
'warning' => ['warning', 'alarm'],
'info' => ['info', 'notice', 'alert'],
],
This option maps your framework's flash message types to PHPFlasher notification types:
-
The keys (
success
,error
, etc.) are PHPFlasher notification types - The values are arrays of framework flash types that map to each PHPFlasher type
This allows PHPFlasher to convert your existing flash messages automatically.
'filter' Option
'filter' => [
'limit' => 5,
// You can add more filter criteria here
],
This option lets you control which notifications are displayed:
-
limit
: Maximum number of notifications to show at once
This is helpful to prevent overwhelming users with too many notifications at once.
'presets' Option
'presets' => [
'entity_saved' => [
'type' => 'success',
'title' => 'Entity saved',
'message' => 'Entity saved successfully',
],
'payment_failed' => [
'type' => 'error',
'title' => 'Payment failed',
'message' => 'Your payment could not be processed',
],
],
Presets are reusable notification templates that you can use throughout your application:
- Define common notifications once in your configuration
-
Use them by name with
flash()->preset('entity_saved')
This reduces code duplication and ensures consistent messaging.
Practical Configuration Examples
Let's look at some real-world examples to understand how these configuration options work together:
return Configuration::from([
'default' => 'toastr',
'main_script' => '/vendor/flasher/flasher.min.js',
'styles' => [
'/vendor/flasher/flasher.min.css',
],
'options' => [
'timeout' => 5000,
'position' => 'top-right',
],
'inject_assets' => true,
'translate' => true,
'flash_bag' => [
'success' => ['success'],
'error' => ['error', 'danger'],
'warning' => ['warning'],
'info' => ['info'],
],
]);
return Configuration::from([
'default' => 'sweetalert',
'main_script' => 'https://cdn.example.com/flasher.min.js',
'styles' => [
'https://cdn.example.com/flasher.min.css',
],
'options' => [
'timeout' => 8000,
'position' => 'top-center',
],
'inject_assets' => true,
'translate' => true,
'excluded_paths' => [
'api/*',
'admin/reports/*',
],
'flash_bag' => [
'success' => ['success', 'ok'],
'error' => ['error', 'danger', 'fail'],
'warning' => ['warning', 'caution'],
'info' => ['info', 'notice', 'note'],
],
'filter' => [
'limit' => 3,
],
'presets' => [
'user_created' => [
'type' => 'success',
'title' => 'User Created',
'message' => 'The user has been created successfully',
],
'profile_updated' => [
'type' => 'success',
'title' => 'Profile Updated',
'message' => 'Your profile has been updated successfully',
],
'login_failed' => [
'type' => 'error',
'title' => 'Login Failed',
'message' => 'Invalid username or password',
'options' => [
'timeout' => 10000,
],
],
],
]);
Using Configuration in Code
Now let's see how to use these configuration options in your actual code:
Basic Usage
// Using the default adapter and settings
flash()->success('Profile updated successfully!');
// Using a specific adapter
toastr()->error('Something went wrong!');
// Using SweetAlert
sweetalert()->info('Please check your email');
These examples use the global configuration options you've set.
Overriding Options
// Override global settings for a single notification
flash()->option('timeout', 10000)
->option('position', 'bottom-center')
->error('This is important!');
// Using presets
flash()->preset('user_created');
// Using presets with custom message
flash()->preset('login_failed', [
'message' => 'Wrong password. Try again.'
]);
You can override global settings for individual notifications when needed.
Pro Tips for Beginners
Start Simple
Begin with the default configuration and gradually customize options as you become more familiar with PHPFlasher.
Use Presets
Create presets for commonly used notifications to ensure consistency and reduce code duplication.
Test Different Themes
Try different adapters (toastr, sweetalert, etc.) to find the style that best matches your application's design.
Check Console
If notifications aren't working, check your browser's console for JavaScript errors that might help identify configuration issues.
Troubleshooting Common Configuration Issues
Missing Notifications
If notifications aren't appearing:
-
Verify
inject_assets
is set totrue
-
Check that your URL isn't in
excluded_paths
- Make sure the adapter you're using is installed
Script Loading Issues
If you're seeing script errors:
-
Verify your
main_script
path is correct -
Make sure the
styles
paths exist - Check if dependencies like jQuery are loaded first
Config Not Applied
If your config changes aren't taking effect:
- Clear your framework's config cache
- Verify you're editing the correct config file
- Check the syntax of your configuration
Ready to customize your notifications?
PHPFlasher offers endless customization possibilities to make your notifications perfect for your application's needs.
Summary: Key Configuration Options at a Glance
Option | Description | Default |
---|---|---|
default | Default notification adapter | flasher |
main_script | Path to main JavaScript file | /vendor/flasher/flasher.min.js |
styles | CSS files for styling notifications | ['/vendor/flasher/flasher.min.css']
|
inject_assets | Auto-inject assets into HTML | true |
translate | Enable message translation | true |
options.timeout | Default notification display time (ms) | 5000 |
options.position | Where notifications appear on screen | top-right |
Conclusion
PHPFlasher v2 offers a flexible configuration system that makes it easy to customize your notifications exactly how you want them. For beginners, the most important options to understand are:
- default: Choose which notification library you want to use
- inject_assets: Control whether PHPFlasher automatically adds JavaScript/CSS to your pages
- options: Set global defaults for notification behavior (timeout, position, etc.)
- presets: Create reusable notification templates for consistent messaging
Remember that PHPFlasher is designed with sensible defaults, so you don't need to configure everything at once. Start with the basics and gradually expand your configuration as your project grows and your needs evolve.
Final Tips
- Keep your configuration DRY by using global options and presets
- Position notifications consistently throughout your application
- Consider accessibility when setting timeouts (longer for important messages)
- Minimize the number of notifications shown at once to avoid overwhelming users
- Test your notifications on both desktop and mobile devices
With the knowledge you've gained from this guide, you're now ready to customize PHPFlasher to create the perfect notification experience for your users. Whether you're building a simple blog or a complex application, PHPFlasher's configuration options give you the flexibility to implement professional notifications that enhance your user experience.