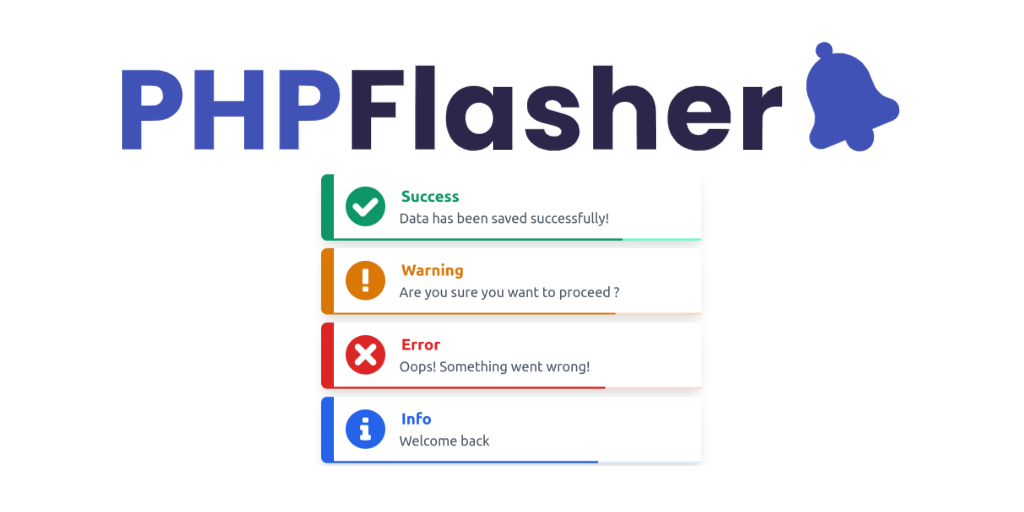
Common PHPFlasher Implementation Mistakes and How to Avoid Them
PHPFlasher makes implementing flash notifications in your PHP applications straightforward, but even the simplest tools can be misused. In this guide, we'll explore common implementation mistakes and provide solutions to help you get the most out of PHPFlasher.
Why This Matters
Proper implementation of flash notifications directly impacts user experience:
- Better User Experience: Correctly implemented notifications provide clear, timely feedback to users.
- Reduced Confusion: Consistent notification behavior helps users understand system status.
- Code Maintainability: Following best practices makes your notification code easier to maintain.
Mistake #1: Incorrect Method Chaining Order
One of the most common mistakes is incorrect method chaining order. In PHPFlasher v2, notification type methods (success()
, error()
, etc.) should be called last in the chain.
Incorrect
// Incorrect order
flash()->success('Profile updated!')
->position('bottom-right')
->timeout(5000);
Correct
// Correct order
flash()->position('bottom-right')
->timeout(5000)
->success('Profile updated!');
Why This Matters
In PHPFlasher v2, configuration methods should come first, and the notification type method should be the final call. This ensures all options are properly applied to the notification.
Mistake #2: Using Outdated Method Names
If you're upgrading from PHPFlasher v1, you might still be using outdated method names.
Outdated (v1)
// v1 method names
flash()->addSuccess('Item created');
toastr()->addInfo('Please check your email');
noty()->addWarning('Your session is expiring');
Current (v2)
// v2 method names
flash()->success('Item created');
toastr()->info('Please check your email');
noty()->warning('Your session is expiring');
Mistake #3: Missing Redirects After Flash Messages
Flash messages are designed to persist across an HTTP redirect. A common mistake is setting a flash message without redirecting afterward.
Incorrect
// Flash message without redirect
public function store(Request $request)
{
// Process the form...
flash()->success('User created successfully!');
// Missing redirect!
return view('users.index');
}
Correct
// Flash message with redirect
public function store(Request $request)
{
// Process the form...
flash()->success('User created successfully!');
// Redirect to display the flash message
return redirect()->route('users.index');
}
When to Skip Redirects
There are legitimate use cases for flash messages without redirects, but they're less common:
- API responses that include flash messages
- AJAX requests with JavaScript-rendered notifications
- Livewire or similar component-based systems
Mistake #4: Forgetting to Auto-Inject Assets in v2
In PHPFlasher v2, assets are automatically injected into your HTML, but some developers still include unnecessary code from v1.
Unnecessary in v2
<!-- Unnecessary in layouts/app.blade.php -->
<!-- Your content -->
@flasher_render
</body>
</html>
Correct for v2
<!-- In layouts/app.blade.php -->
<!-- Just your normal content, no special directive needed -->
</body>
</html>
Assets are automatically injected in v2
Important Configuration Note
Auto-injection is enabled by default in v2 via the inject_assets
configuration option. If you've disabled this feature, you'll need to manually include the assets.
Mistake #5: Inconsistent Notification Positioning
Using different positions for similar types of notifications can confuse users and create a disjointed user experience.
Inconsistent
// Inconsistent positioning
flash()->position('top-right')
->success('Profile updated!');
// Later in another action
flash()->position('bottom-left')
->success('Settings saved!');
// And another action
flash()->position('top-center')
->success('Password changed!');
Consistent
// Consistent positioning
flash()->position('top-right')
->success('Profile updated!');
// Later in another action
flash()->position('top-right')
->success('Settings saved!');
// And another action
flash()->position('top-right')
->success('Password changed!');
Strategic Positioning Guide
Instead of random positioning, adopt a strategic approach:
Success Messages
Use top-right
or bottom-right
for success notifications to be noticeable but not intrusive.
Warnings & Errors
Use top-center
for important warnings and errors to ensure they're seen.
Information
Use bottom-left
or bottom-right
for general information that's less critical.
Mistake #6: Not Utilizing Global Configurations
Repeating the same notification options in multiple places makes your code harder to maintain. PHPFlasher lets you set global configurations:
Repetitive Code
// Repeating the same options
flash()->position('top-right')
->timeout(5000)
->success('Profile updated!');
flash()->position('top-right')
->timeout(5000)
->error('Something went wrong!');
flash()->position('top-right')
->timeout(5000)
->info('New features available!');
Using Global Config
// In config/flasher.php
'options' => [
'timeout' => 5000,
'position' => 'top-right',
],
// In your code
flash()->success('Profile updated!');
flash()->error('Something went wrong!');
flash()->info('New features available!');
Mistake #7: Overloading Users with Notifications
Showing too many notifications at once can overwhelm users and diminish the importance of each message.
Too Many Notifications
public function checkout()
{
// Too many notifications at once
flash()->success('Order placed successfully!');
flash()->info('You will receive an email confirmation.');
flash()->info('Your items will be shipped tomorrow.');
flash()->success('You earned 100 reward points!');
flash()->info('Check out our new products!');
return redirect()->route('orders.confirmation');
}
Prioritized Notifications
public function checkout()
{
// Only the most important notifications
flash()->success('Order placed successfully! You will receive an email confirmation.');
// Conditionally show only if it's their first order
if ($isFirstOrder) {
flash()->info('You earned 100 reward points!');
}
return redirect()->route('orders.confirmation');
}
Notification Prioritization Checklist
When deciding which notifications to show, consider:
- Is it actionable? Notifications requiring user action should take priority.
- Is it time-sensitive? Urgent information should be shown immediately.
- Is it contextually relevant? Show information related to the user's current task.
- Can it be combined? Merge related messages when possible.
- Could it be displayed elsewhere? Consider inline messages for non-critical information.
Mistake #8: Ignoring Accessibility
Many developers forget to consider accessibility when implementing notifications, making their applications less usable for people with disabilities.
Poor Accessibility
- Using colors alone to convey message type
- Setting timeouts too short for important messages
- Not providing clear, descriptive messages
- Using low contrast color combinations
Accessible Approach
- Using icons and colors together to indicate message type
- Setting longer timeouts for important messages (8-10 seconds)
- Providing clear, specific message content
- Using high contrast colors that meet WCAG standards
Accessibility Tip
For critical errors or success messages that require acknowledgment, consider using timeout(0)
to prevent auto-dismissal, ensuring users have enough time to read the message.
Mistake #9: Library Conflicts
Using multiple JavaScript libraries that manipulate the DOM can sometimes cause conflicts with PHPFlasher.
Common Sources of Conflicts
-
Multiple Notification Libraries
Having both Toastr and SweetAlert loaded directly alongside PHPFlasher can cause conflicts.
Solution: Use PHPFlasher's adapters instead of loading libraries separately.
-
CSS Collisions
Your application's CSS might override PHPFlasher's styles.
Solution: Use more specific selectors in your CSS or customize PHPFlasher's theme.
-
JavaScript Errors in Other Scripts
Errors in other scripts might prevent PHPFlasher from initializing properly.
Solution: Check your browser console for errors and fix them.
Mistake #10: Not Testing on Mobile Devices
Notifications that look great on desktop might be problematic on mobile devices if not properly tested.
Mobile Issues
- Notifications covering important UI elements
- Text that's too small to read on mobile screens
- Notifications that are too wide for the mobile viewport
- Touch targets that are too small for comfortable interaction
Mobile Solutions
-
Use
top-center
orbottom-center
positions on mobile - Keep messages concise to avoid excessive wrapping
- Use responsive width settings that adapt to screen size
- Test on real mobile devices or accurate emulators
Want to avoid these mistakes in your projects?
PHPFlasher provides an elegant, powerful solution for notifications with minimal potential for implementation errors.
Bonus: Using Presets for Consistent Notifications
In PHPFlasher v2, you can define presets in your configuration to ensure consistent notifications across your application:
'presets' => [
'entity_saved' => [
'type' => 'success',
'message' => 'Entity saved successfully',
'options' => [
'timeout' => 3000,
'position' => 'top-right',
],
],
'entity_deleted' => [
'type' => 'success',
'message' => 'Entity deleted successfully',
'options' => [
'timeout' => 3000,
'position' => 'top-right',
],
],
'validation_error' => [
'type' => 'error',
'message' => 'Please check the form for errors',
'options' => [
'timeout' => 5000,
'position' => 'top-center',
],
],
],
Then in your controllers, you can use these presets like this:
// Use the preset directly
flash()->preset('entity_saved');
// Or customize the preset message
flash()->preset('entity_saved', ['message' => 'User saved successfully']);
// Or with placeholders in your preset message
// If your preset has: 'message' => 'Entity :name saved successfully'
flash()->preset('entity_saved', ['name' => 'User']);
Recap: Top 10 PHPFlasher Implementation Mistakes
-
1
Incorrect Method Chaining Order
Always put notification type methods (success, error, etc.) at the end of the chain.
-
2
Using Outdated Method Names
Use
success()
instead ofaddSuccess()
, etc. in v2. -
3
Missing Redirects After Flash Messages
Flash messages are designed to survive redirects; use them appropriately.
-
4
Forgetting Auto-Inject Assets Feature in v2
No need for
@flasher_render
in v2 as assets are auto-injected. -
5
Inconsistent Notification Positioning
Use consistent positioning for similar notification types.
-
6
Not Utilizing Global Configurations
Set common options in the configuration file to reduce repetition.
-
7
Overloading Users with Notifications
Show only the most important notifications to avoid overwhelming users.
-
8
Ignoring Accessibility
Ensure notifications are accessible to all users, including those with disabilities.
-
9
Library Conflicts
Be mindful of JavaScript library conflicts that might affect PHPFlasher's functionality.
-
10
Not Testing on Mobile Devices
Ensure notifications look and function properly on mobile devices.
Conclusion
PHPFlasher is a powerful tool for implementing notifications in your PHP applications. By avoiding these common mistakes, you can ensure your notifications are effective, consistent, and enhance the user experience rather than detract from it.
Remember that notifications are a communication tool between your application and your users. Keep them clear, relevant, and appropriately timed, and they'll contribute to a positive user experience.